There are many ways to make custom changes in WordPress. However, one of the most important to understand is using a child theme.
Let’s see how it works.
What is a WordPress child theme?
WordPress child themes allow you to safely add custom code to your “parent” theme.
For example, say I have the “Twenty Twenty-Two” theme activated and I want to add custom CSS to change the default buttons.
I could add custom CSS directly to the theme’s stylesheet, but this would be a bad practice. The reason? File changes made directly to a parent theme will be deleted after the theme is updated. Not good! 😰
So instead, the main benefit of adding customizations to a child theme is that they will not disappear after updating your parent theme.
You get to inherit all the great stuff about your theme, plus safely make any customizations that you want.
Cool huh? 😏
How do I make a WordPress child theme?
First, you need access to your WordPress files with something called FTP access. You can usually find this inside your webhost dashboard. If you’re unsure, just contact them and they’ll get you setup.
Step 1. Create the child theme folder
Assuming you have access to your website files, begin by making a new folder next to your parent theme.
Once you have the folder created, you’ll need to give it a name. A good rule of thumb is to use the same name but with a “-child” suffix at the end.
For example, I’ll name my child theme twentytwentytwo-child since my parent theme is twentytwentytwo.
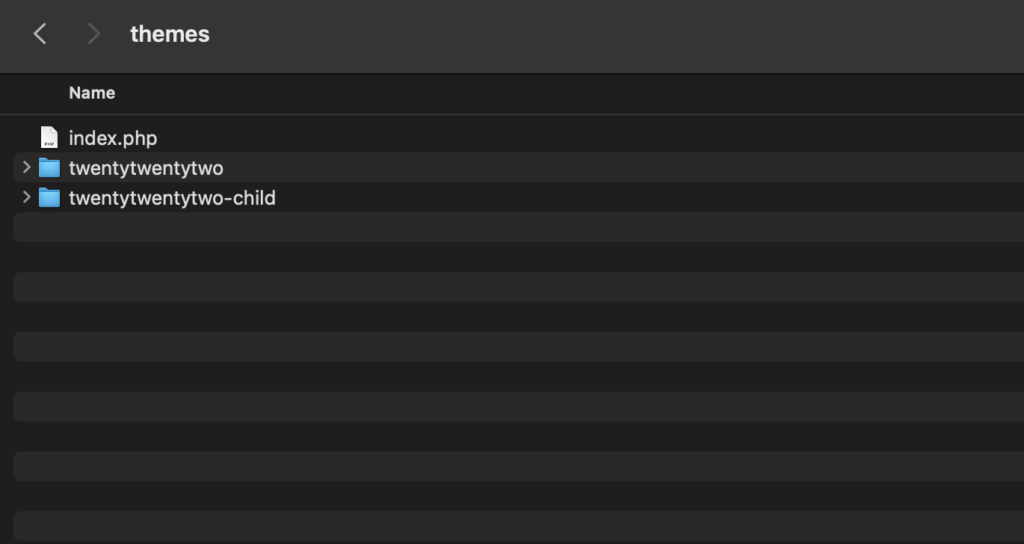
There are two files required to make a child theme: style.css
and functions.php
. These two files are necessary to connect our child theme to the parent theme. It’s pretty simple, so let’s do that now.
Step 2. Add the style.css file
As mentioned, we need to create a file called style.css
. This file will tell our child theme which parent theme to inherit.
Inside the child theme folder, create a file called style.css
and then copy / paste the below code inside of it.
/*
Theme Name: Twenty Twenty-Two Child
Template: twentytwentytwo
*/
What you added are called comment headers. They tell WordPress that we’re using a child theme and which parent theme we should inherit.
We’re telling WordPress that we want to inherit the Twenty Twenty-Two parent theme with the “Template” header comment. The value of this comment corresponds to the name of the parent theme directory. Your theme will probably be different, so make sure to change this value.
However for this to actually work, we need to enqueue our newly created style.css
file.
Let’s do this now.
Step 3. Add the functions.php file
Like we did with style.css
, we’ll now create another file called functions.php
. Make this file at the same level as style.css like this:
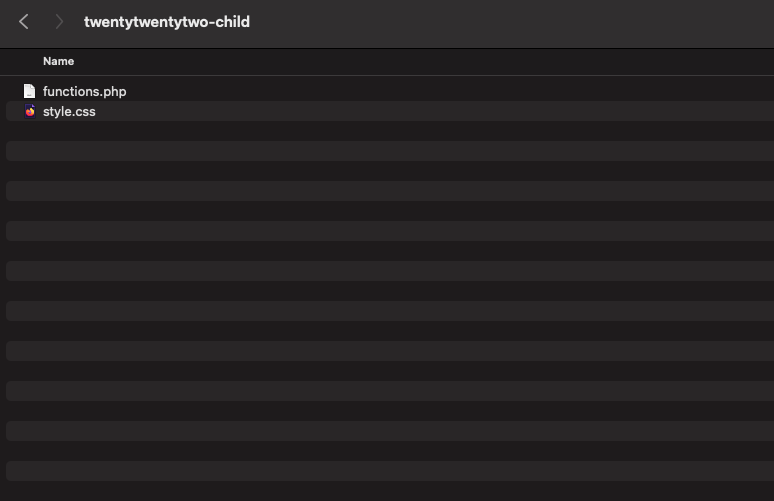
Once you have this file created, copy / paste the below code inside:
<?php
function child_styles() {
wp_enqueue_style( 'child-theme-css', get_stylesheet_directory_uri() . '/style.css', []);
}
add_action( 'wp_enqueue_scripts', 'child_styles', 99 );
This imports (or enqueues) our child theme’s style.css
file.
Congrats! 🙌 You’re close to having a working child theme. Let’s finish the process.
Step 4. Activate your new child theme
Now that we have our child theme created, the last step is to activate it.
Open the dashboard and click Appearance - Themes
. You should see your child theme listed there without a screenshot. Simply click activate and you’re done!
There are more things you can customize with your child theme, but this is technically all you need. For a full list of child theme options, have a look at the official WordPress docs.
Using a child theme for ShopWP changes
ShopWP provides many opportunities for customization. For example, if you open the settings you’ll see different options for products, collections, cart, etc.
However, this is just the tip of the iceberg! There are tons of options for customizing core features of the plugin with small code snippets.
Where do these code snippets go? You guessed it. The child theme!
So let’s see how this works. 👇
Using JavaScript hooks
All the JavaScript hooks we provide require a child theme to use. The reason, is that the JavaScript you write needs to run after ours. The only way to ensure this happens is to import (or enqueue) your JavaScript at the right point. Also keep in mind that some of our hooks require ShopWP Pro.
To begin, create a new file in your theme called shopwp-scripts.js
.
You can keep it empty for now.
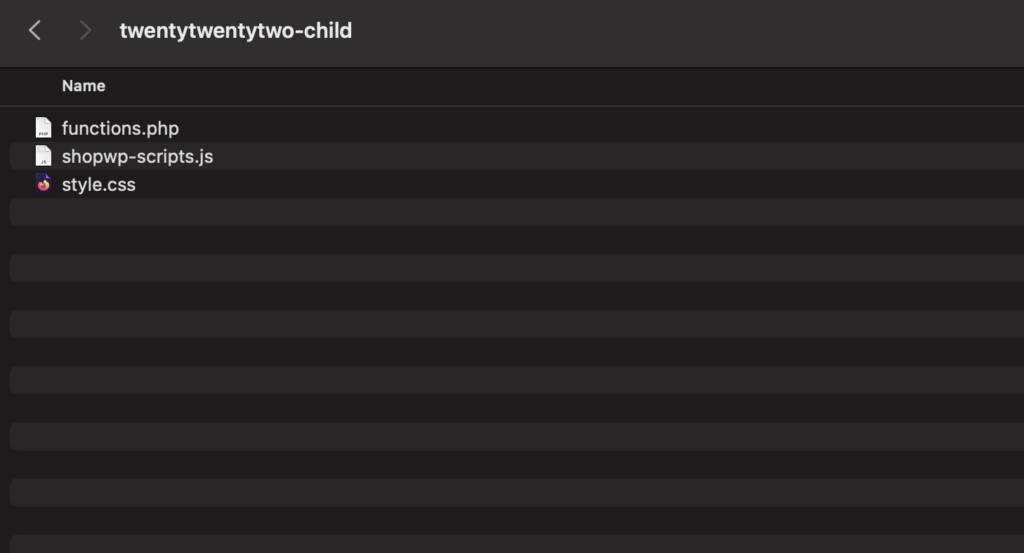
Next, open the functions.php
file and copy / paste the below snippet at the very bottom below everything else, like this:
function theme_assets() {
wp_enqueue_script( 'shopwp-scripts', get_stylesheet_directory_uri() . '/shopwp-scripts.js', ['shopwp-public'], '', true);
}
add_action('wp_enqueue_scripts', 'theme_assets');
Take a look at the code you just added. Do you see the shopwp-public
part? This is the most important piece of the puzzle. This tells WordPress to load your custom JavaScript after ShopWP.
So now at this point, any hook you at to your shopwp-changes
file should work. You can test this by adding the below JavaScript to the file:
wp.hooks.addFilter('product.titleText', 'shopwp', function (productTitle, productPayload) {
return productTitle + ' plus custom text';
});
After saving the file, reload your products page and you should see the text “plus custom text” appended to every product title.
This indicates that your child theme is loading the custom JavaScript, and the ShopWP hook is running correctly.
Nice job! 👊
Using PHP hooks
In order to use the ShopWP PHP hooks, you’ll need to add the relevant code snippets to your child theme’s functions.php
file.
For example, if you want to change the default color of all product title’s, you can leverage the shopwp_products_default_payload_settings
filter like this:
add_filter('shopwp_products_default_payload_settings', function($payloadSettings) {
$payloadSettings['title_color'] = 'green';
return $payloadSettings;
});
That’s it!
Keep in mind that some of our PHP hooks require ShopWP Pro. If you’re unsure, look for the “pro-only” label when viewing the docs.
Best practices when using a child theme
As you’ve seen, creating a WordPress child theme is fairly straight forward. However there are some best practices to keep in mind.
Naming conventions
First, it’s a good idea to follow the standard convention when naming your child theme folder. The best practice is to use the name of the parent theme with -child
appended to the end.
So if your parent theme folder is called twentytwentytwo
, then name your child theme folder twentytwentytwo-child
. Following this trend helps all WordPress developers quickly understand what’s going on, should you ever hand the project over.
Always consider using a child theme
You may be wondering, should I just always use a child theme? My answer would be yes. Unless your theme is completely built from scratch, it’s usually a good idea to automatically create a child theme anytime you purchase or install a new WordPress theme.
One of the nice things about many of the popular themes is that they will usually provide a drag and drop child theme in your purchase bundle. So instead of manually creating it yourself, you can just drag it into your wp-content/themes
folder and be done.
Never edit your parent theme directly
I think it goes without saying but just to emphasis again, you should never directly edit your parent theme. If you do, you will lose your changes.
Some people may rationalize and tell themselves that it’s just one simple change, what could hurt? I’m low on time, I just need to get this one thing fixed.
While this may be true, I guarantee you’ll forget about that change in two months. Then you’ll update the parent theme and cause yourself more problems.
Overall, a child theme is a must have for any WordPress site owner that’s interested in making even small customizations to their site.
I hope this article was helpful, good luck!